Introduction
As we all know, Drupal 8 is shipped with pluggable architecture which powers many more features in than its previous versions, like blocks, field widget, field formatter, etc. Typically plugins are small pieces of functionality that are swappable. Plugins that have similar functionality are of the same plugin type. You can read more about plugins here.
Let’s look at creating a Drupal custom formatter plugin that can parse data from an uploaded CSV file. We’ll also go through the various functions required to implement a custom plugin.
Code discussed here is shared in this GitHub repository: https://github.com/mohit-rocks/csv_field_formatter
Step 1: Create an info file for the module
Create a basic info.yml file, which is required for the module.
name: Field Formatter Demo module type: module description: 'Provides sample code to parse CSV content using field formatter' package: Examples core: 8.x |
Step 2: Create related files and class
In this step we will be creating a plugin class. As we have created a file field to upload CSV files, we need to extend the FileFormatterBase class.
Typically, Drupal core plugins are defined by specific modules. For example, file field related plugins are stored in the file module. You can also refer to the “Drupal\Core\Field\Plugin\Field\FieldFormatter” namespace to find related plugins.
Now, we’ll create a “src/Plugin” folder inside our module, in which we typically store all plugin related files. In case of the field formatter plugin, our file should be stored inside the “src/Plugin/Field/FieldFormatter” directory. Below is our code for class declaration and annotated plugin definition.
use Drupal\Component\Utility\Html; use Drupal\Core\Field\FieldItemInterface; use Drupal\Core\Field\FieldItemListInterface; use Drupal\file\Plugin\Field\FieldFormatter; use Drupal\Core\Form\FormStateInterface; use Drupal\file\Plugin\Field\FieldFormatter\FileFormatterBase; /** * Plugin implementation of the 'csvdisplay_field_formatter' formatter. * * @FieldFormatter( * id = "csvdisplay_field_formatter", * label = @Translation("CSV Display field formatter"), * field_types = { * "file" * } * ) */ class CSVDisplayFieldFormatter extends FileFormatterBase { |
Again, note that we are extending the “FileFormatterBase” class, as we are implementing file field plugins.
Step 3: Implement the settings form
We know that field formatter plugins can act on user-specified configurations as well, so in this step we will create a configuration form. In this form, we will specify a column name, i.e. the column name from the CSV file we want to display.
For ease of use, we have provided another checkbox that will decide whether we should display the CSV file as well. So the entire section of code should look something like this:
/** * {@inheritdoc} */ public static function defaultSettings() { return [ 'csv_column' => 'first_name', // Column name that you want to display. 'show_file' => 0, ] + parent::defaultSettings(); } /** * {@inheritdoc} */ public function settingsForm(array $form, FormStateInterface $form_state) { $form = parent::settingsForm($form, $form_state); $form['csv_column'] = [ '#title' => $this->t('Column name from CSV file.'), '#description' => $this->t('The column name that you want to render from the uploaded CSV file. It is expected that all uploaded CSV files should be of the same format.'), '#type' => 'textfield', '#default_value' => $this->getSetting('csv_column'), ]; $form['show_file'] = [ '#title' => $this->t('Display CSV file.'), '#description' => $this->t('Check this checkbox to display the CSV file in generic file formatter.'), '#type' => 'checkbox', '#default_value' => $this->getSetting('show_file'), ]; return $form; } |
Here, we have implemented the default settings function as well, so that upon installation, the field formatter will have default column name value and file display settings. Here, I am using the column name “first_name” from my CSV file.
Step 4: Displaying a summary for field formatter settings
After setting field formatter values, we can display them in summary items.
The code below will display the summary.
/** * {@inheritdoc} */ public function settingsSummary() { $summary = []; $settings = $this->getSettings(); $summary[] = $this->t('Column to display: ') . $settings['csv_column']; $summary[] = ($settings['show_file'] == 1) ? $this->t('Display file : Yes') : $this->t('Display file : No'); return $summary; } |
Step 5: Displaying and rendering data
To show data in a field, we need to implement the “viewElements()” method. In this method we can retrieve data and render data. You can refer to “viewElements()”.
In this function, with the help of a few PHP functions, we are reading data from the CSV file and rendering data as an items list.
So that’s how you can leverage Drupal 8’s plugin API to create a field formatter plugin and read data from a CSV file’s columns. If you found this post helpful or if you have any questions, do leave a comment below.
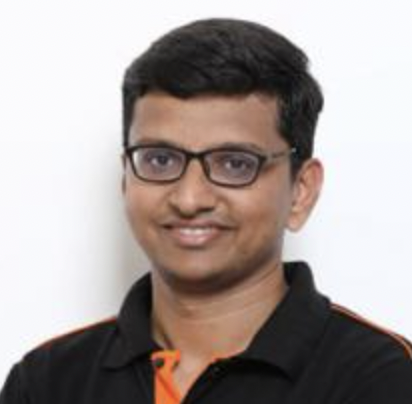
Leave us a comment