Introduction
There are many things that developers should avoid during Drupal development. There are a hundred ways to make a feature work, but not all those ways are equally good, and that makes all the difference between developers. Developers should write better code because code needs to be maintainable.
While working on my latest task, I received some valuable feedback from a peer which helped me learn how to write cleaner code and avoid common mistakes. They suggested that I share these learnings with the community—and that’s how I began writing this post.
So let's discuss a few things to consider for writing clean code.
Declare meaningful names for functions and variables.
Variables: Variable names should reveal their intent. They should explain the variable’s exact contents, why it exists and what it does.
Methods: The name must specify the exact action of the method and meaningful names for method parameters, so that it documents itself.
This is well documented in drupal.org, here and here.
Replace your loops using array_map().
We can replace loops with a call to the array_map function.
This allows you to define a function which will be executed over each element in the array. It creates a new array that contains the mapped entries.
array_map accepts at least two parameters: a callable and an array. It iterates through each array value and passes it to the given callable.
The callable applies changes to the array value and returns it back to array_map.
The big difference between foreach() and array_map() is that the array_map() method returns a new array, while foreach doesn’t return anything.
Let's try this in Drupal.
Working with foreach() loop
$node - an array of node
$field_name = 'field_contribution_type'
$reference_type_field = ‘name’
Introducing mapping
The array_map() method is much clearer than a loop.
The code is more readable, understandable, and communicates the intention properly.
Why use the array function?
I prefer the map function because it’s cleaner.
Of course, there are a few drawbacks of the map function as well as basic loops. They both do not provide complete alternatives of each other.
We can find many articles that debate the speed of foreach and map function. In terms of speed, array functions are a little bit slower than basic loops. But I think this does not have a huge impact on performance. There are a lot of other factors that are bigger considerations than map functions when we’re talking about performance improvement.
Finally, I can say that one of the reasons for using the basic loops method is familiarity. Usability is completely depends on the individual—both have their own potential benefits. And if you haven't tried the map function before, that’s something new you can learn and use.
Avoid else when it’s not needed.
This makes the code cleaner, and helps avoid unnecessary coding and execution.
Let's try this with code snippets.
While nothing seems wrong with this, we can avoid else in this approach.
A better approach might be:
Use relevant services
In Drupal 8, a service is a PHP object that performs some sort of global task. This means that services are separated from the specifics of “why” that task would need to be performed and concentrate solely on “how” to perform that task.
The services exposed by Drupal 8 core can be found in /core/core.services.yml. If you need functionality from the Drupal 8 system, either from core or contribute, you'll be requesting services.
Using dependency injection, all you need to do is declare your dependency on them in your own code, and then make use of them when your class is instantiated.
Let's take an example where we need user details and node details. We can use different services for loading details.
Eg:
Instead of loading different services, we can get user and node details using the same services.
We can load a service using the create method. This is a factory method that returns a new instance. The create method is part of Drupal\Core\DependencyInjection\ContainerInjectionInterface. It allows controllers to be instantiated with services.
__contruct method uses services that are loaded by the create method, and stores them in properties of the class.
For more information, visit https://www.drupal.org/node/2133171.
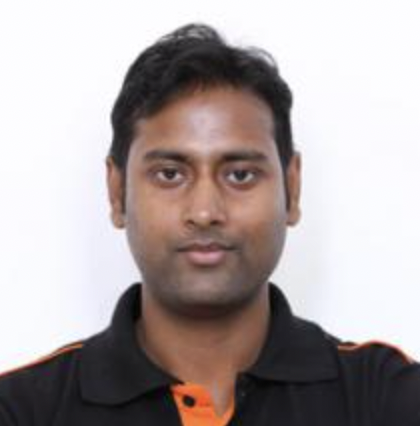
Sumit Kumar, PHP/Drupal Engineer - L2
Utopian lover of theatre and showbusiness. Prefers being on stage when he's not online.
Leave us a comment