Introduction
In the first part of this series, we discussed how to set up and work with the Postman API testing tool, and we ran a basic test.
In this part, we will discuss the technical points which would be helpful to developers and testers in order to make dynamic API calls. This would be helpful with API testing via Postman.
Below are the JSON snippets which would be helpful to create test scenarios. For basic execution, please refer to Part 1 of API Testing with Postman.
1. To convert the response in JSON format
var responseJSON;
try {
responseJSON = JSON.parse(responseBody);
tests['response is valid JSON'] = true;
}
catch (e) {
responseJSON = {};
tests['response is valid JSON'] = false;
}
2. To set the variable value into environment
Response from the POST or GET “API” is:
"data": {
"id": 470595271655486,
"type": "staffSubstitution",
"accountId": 8800387990610,
"status": "A"
}
postman.setEnvironmentVariable(key,
For example:
postman.setEnvironmentVariable("environment variable value", responseJSON.data.id);
3. To set the variable as global
postman.setEnvironmentVariable(key, value)
For example:
postman.setGlobalVariable("Global variable value ", responseJSON.data.id);
4. To check if the response has the data parameter or not
Response from the POST or GET “API” is:
"data": {
"id": 470595271655486,
"type": "staffSubstitution",
"accountId": 8800387990610,
"status": "A"
}
tests['response json contain Data] = _.has(responseJSON, data);
5. To check the length of the attribute in the response
Response from the POST or GET “API” is:
"data": {
"id": 470595271655486,
"type": "staffSubstitution",
"accountId": 8800387990610,
"status": "A"
}
tests[' attribute count under the data '] = (responseJSON.payload && Object.keys(responseJSON.data).length === 4);
6. To check the status of the attribute
Response from the POST or GET “API” is:
"data": {
"id": 470595271655486,
"type": "staffSubstitution",
"accountId": “8800387990610”,
"status": "A"
}
tests['Data must have accountId'] = (responseJSON.data && responseJSON.data.accountId === "8800387990610");
7. To check if the response has a message or not
Response from the POST or GET “API” is:
{
"message": "Organization has been created successfully.",
}
tests['response has message'] =( responseJSON.message == "Organization has been created successfully.");
8. To check the status code
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
9. To check the Successful POST request status code
pm.test("Successful POST request", function () {
pm.expect(pm.response.code).to.be.oneOf([201,202]);
});
10. To clear the environment variable
postman.clearEnvironmentVariable("key");
11. To check JSON value
Response from the POST or GET “API” is:
"data": {
"id": 470595271655486,
"type": "staffSubstitution",
"accountId": “8800387990610”,
"status": "A",
“value”:130
}
Part 3 of this series covers the technical aspects of command line integration with Newman and Postman.
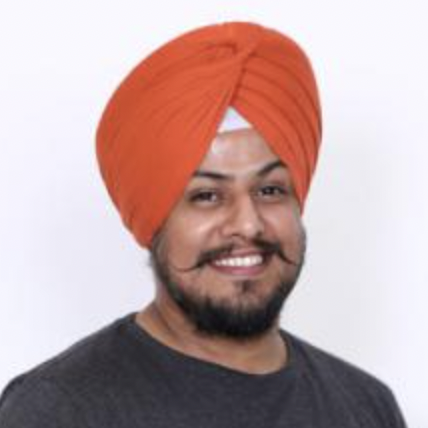
Jaspreet Singh, Senior Project Manager
Nickname: Jazz. Family man, obsessed with watching, playing, reading about cricket—if you play a round of "snooker" with him, prepare to lose.
Leave us a comment